Quickstart
This page will get you up and running quickly so you can start playing with kwerc. For setting up a real web app with a background service, database, TLS, ramdisk, and CDN, see Installation.
Installation
We use Debian but kwerc should work nearly anywhere with C & Go compilers.
sudo apt install build-essential golang-go
git clone https://github.com/kwerc/kwerc
cd kwerc
make
Running kwerc
./bin/cgd -c kwerc/es/kwerc.es
You can now visit your site at http://127.0.0.1:42069. Try registering a user account and logging in.
Your first page
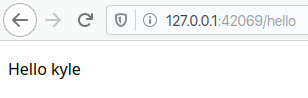
Files under kwerc/site/
will be served on the web, i.e. kwerc/site/somedirectory/somefile.html
→ http://example.com/somedirectory/somefile
.
.html
files are served as you would expect. kwerc also supports .tpl
template files which can combine HTML and es code. Both .html
and .tpl
files will be inlined in the <body>
of the master template (kwerc/tpl/master.tpl
) by default, so you don't need to worry about defining <html>
, <head>
, or <body>
.
Let's create a page at kwerc/site/hello.tpl
. Go ahead and make this file with the following contents:
<p>Hello
% if {logged_in} {
% echo $logged_user
% }
<p>
Lines beginning with %
are interpretted by es. Here, if the user is logged in, we greet them by their username. Otherwise, we just say Hello.
Test it out at http://127.0.0.1:42069/hello.
Your first form
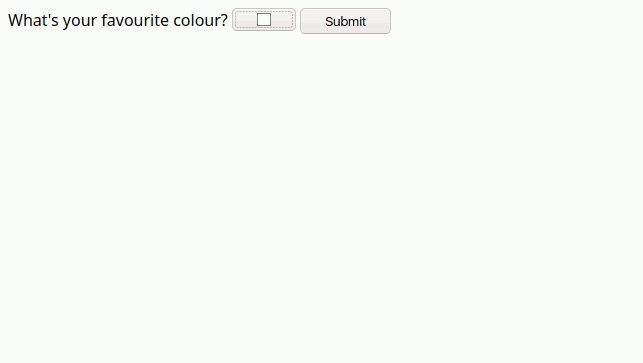
Let's try something a little fancier. Create kwerc/site/colour.tpl
:
<form action="" method="POST">
<label for="colour">What's your favourite colour?</label>
<input type="color" name="colour" id="colour" required>
<button type="submit">Submit</button>
</form>
We could process this form input right in our .tpl
, but for the sake of organization kwerc also lets you put es code in a .es
file. This will be executed before your .tpl
. Create kwerc/site/colour.es
:
require_login
if {!~ $REQUEST_METHOD POST} { return 0 }
if {!~ $p_colour '#'[0-9a-f][0-9a-f][0-9a-f][0-9a-f][0-9a-f][0-9a-f]} {
throw error 'Invalid colour'
}
echo $p_colour > db/users/$logged_user/colour
First we run require_login
. If the user isn't logged in, this will prevent them from seeing this page and redirect them to the login page. Once they're logged in, they'll automatically be redirected back to this page.
If this isn't a POST request (i.e. a form submission), we exit colour.es
and continue on to colour.tpl
.
Next, we validate the colour input to make sure it's a proper hex code. POST arguments are automatically stored in variables $p_XXX
where XXX
is the argument name. (Query arguments are also stored in $q_XXX
.) If the input is invalid, we'll throw an error which will be displayed to the user.
Finally, if we've made it this far, we have a valid input and we store it in our database under the user's directory. A plain file tree is the easiest way to store data with kwerc and allows you to take advantage of es's powerful I/O redirection, but files can be slow so we support real databases too.
Now let's update colour.tpl
to do something with our user's favourite colour:
<form action="" method="POST">
<label for="colour">What's your favourite colour?</label>
<input type="color" name="colour" id="colour" required>
<button type="submit">Submit</button>
</form>
% if {logged_in && test -s db/users/$logged_user/colour} {
<style>
body {
background-color: %(`{cat db/users/$logged_user/colour}%);
}
</style>
% }
If the user has set a valid favourite colour, the page background will use it. Beautiful.
Those are the basics of kwerc! Start playing around or continue for more documentation.